Are you a Python developer looking for a job? The 40 Python interview questions below contain basic to expert level interview questions asked by recruiters. Each question is accompanied by an answer so that you can prepare for developer job interviews in a short time.
We have compiled this list after accompanying dozens of technical interviews in top-notch tech companies. Often, these questions and concepts are used in a Python developer’s daily programming work. But these are most helpful when an interviewer is trying to test your deep knowledge of Python.
The difficulty rating on these questions varies from junior level software developers to senior software developers:
- Python interview questions for beginners
- Python interview questions for intermediate developers
- Python interview questions for senior developers
As you read through these Python interview questions, mark the ones you can not answer by yourself. Then, in the second pass, only go through the questions you could not answer in the first place. After going through this list two to three times, you will be well prepared to face a technical developer interview on Python for! There are numerous jobs waiting for you.
Python interview questions for beginners
1. What are the benefits of using Python?
- Efficient: Python is very efficient in memory management. For a large data set like Big Data, it is much easier to program in Python.
- Faster: Though Python code is interpreted, still Python has very fast performance.
- Wide usage: Python is widely used among different organizations for different types of projects. Due to this wide usage, there are thousands of add-ons available for use with Python.
- Easy to learn: Python is quite easy to learn. This might be the biggest advantage of using Python. Complex tasks can be easily implemented in Python.
2. What is the use of PEP 8 in Python?
PEP 8 is a style guide for Python code. This document provides the coding conventions for writing code in Python. The coding conventions are about indentation, formatting, tabs, maximum line length, imports organization, line spacing etc. We use PEP 8 to bring consistency to our code which makes it easier for other developers to read the code.
3. What is the difference between a tuple and list in Python?
In Python, tuples and lists are built-in data structures. Some of the differences between Tuple and List are as follows:
- Syntax: A Tuple is enclosed in parentheses. A List is enclosed in brackets.
- Mutable: Tuple is an immutable data structure. Whereas, a list is a mutable data structure.
- Size: A tuple takes much less space than a list in Python.
- Performance: Tuple is faster than a list in Python. So it gives us good performance.
4. What is the difference between list and dictionary data types in Python?
Main differences between List and Dictionary data types in Python are as follows:
- Syntax: In a list we store objects in a sequence. In a dictionary we store objects in key-value pairs.
- Reference: In a list we access objects by index number. lt starts from 0 index. In a dictionary we access objects by keys specified at the time of dictionary creation.
- Ordering: In a list, objects are stored in an ordered sequence. In a dictionary, objects are not stored in an ordered sequence.
- Hashing: In a dictionary, keys have to be hashable. In a list there is no need for hashing.
5. What are the built-in types available in Python?
Same of the built-in types available in Python are as follows:
Numeric types: These are the types used to represent numbers in Python.
- int: lt is used for Integers.
- lang: lt is used for very large integers of non-limited length.
- float: lt is used for decimal numbers.
- complex: This one is for representing complex numbers.
Sequence types: These types are used to represent sequences of characters or objects.
- str: This is similar to String in Java. lt can represent a sequence of characters.
- bytes: This is a sequence of integers in the range of 0-255.
- byte array: Works like bytes, but mutable (see below); only available in Python.
- list: This is a sequence of objects.
- tuple: This is a sequence of immutable objects.
Sets: These are unordered collections.
- set: This is a collection of unique objects.
- frozen set: This is a collection of unique immutable objects.
Mappings: This is similar to a Map in Java.
- dict: This is also called hashmap. lt has a key value pair to store information by using hashing.
6. How will you concatenate multiple strings together in Python?
We can use following ways to concatenate multiple string together in Python:
Use + operator:
E.g.
>>>firstname="John"
>>>lastname= "Ray"
>>>print firstname+lastname
JohnRay
Use join function:
E.g.
>>> ".join{['John','Ray'])
'JohnRay'
7. What is the use of the pass statement in Python?
The pass statement is used as a placeholder for future code. Nothing happens when it executes, but you avoid getting an error when empty code is not allowed. Empty code is not allowed in loops, function definitions, class definitions, or in if statements.
8. What is the use of slicing in Python?
Slicing is a string operation for getting a substring, or some part of a list. In Python, a string (say text) begins at index 0, and the nth character stores at position text[n-1]. Python can also perform reverse indexing, i.e., in the backward direction, with the help of negative numbers. In Python, the slice() is also a constructor function which generates a slice object. The result is a set of indices mentioned by range(start, stop, step). The slice() method allows three parameters. 1. start – starting number for the slicing to begin. 2. stop – the number which indicates the end of slicing. 3. step – the value to increment after each index (default = 1).
9. What is the difference between an iterator and iterable in Python?
Iterators in Python are array-like objects which allow moving on the next element. We use them in traversing a loop, for example, in a “for” loop. Python library has a number of iterators. For example, a list is also an iterator and we can start a for loop over it. The collection type like a list, tuple, dictionary, and set are all iterable objects whereas they are also iterable containers which return an iterator while traversing.
10. What is the lambda expression in Python?
A lambda expression in Python is used for creating an anonymous function that can accept any number of arguments, but can only have a single expression. Lambda does not contain block of statements. It does not contain return statements. It is generally used in situations requiring an anonymous function for a short time period.
11. What is None in Python?
None keyword represents the null values in Python. Boolean equality operation can be performed using these NoneType objects.
12. What is the use of the // operator in Python?
Python provides the // operator to perform floor division of a number by another. The result of the // operator is a whole number quotient that was divided by the left number with the right number. It can be also used floordiv(a,b).
13. What is a module in Python?
A module is a file written in Python containing Python code with import statements, classes, functions etc. Modules can be imported into another Python file. With modules functionality of applications can be divided into smaller parts to structure a program. The folder of a Python program is a package of modules. A package can have modules or subfolders.
14. How can we create a dictionary with an ordered set of keys in Python?
In a normal dictionary in Python, there is no order maintained between keys. To solve this, the OrderDict class can be used. The class is available since version 2.7.
15. Python is an object-oriented programming language or a functional programming language?
Python is an object-oriented programming language. However, Python can be treated as procedural as well as structural language.
16. What is the difference between the append() and extend() methods of a list in Python?
In Python, the append() and extend() methods are used to add elements at the end of a list.
- append(element): Adds the given element at the end of the list.
- extend(another list): Adds the elements of another list at the end of the list.
17. What are Errors and Exceptions in Python programs?
Errors are coding issues in a program which may cause it to exit abnormally.Exceptions happen due to the occurrence of an external event which interrupts the normal flow of the program.
Python interview questions for intermediate developers
18. How will you improve the performance of a program in Python?
There are many ways to improve a Python program. Some of these are as follows:
- Data Structure: We have to select the right data structure for our purpose in a Python program.
- Standard Library: Wherever possible, we should use methods of standard libraries. Methods implemented in standard libraries have much better performance than user implementations.
- Abstraction: At times, a lot of abstraction and indirection can cause slow performance of a program. We should remove the redundant abstraction in our source code.
- Algorithm: Use of the right algorithm can make a big difference in a program. We have to find and select the suitable algorithm to solve problems with high performance.
19. What is pickling in Python?
Pickling is a process by which a Python object hierarchy can be converted in a byte stream. The reverse operation of pickling is unpickling. Python has a module named pickle. This module has the implementation of a powerful algorithm for serialization and deserialization of Python object structures. Some people also call pickling as serialization or marshalling. With serialization we can transfer Python objects over the network. It is also used in persisting the state of a Python object. We can write it to a file or database.
20. How does memory management work in Python?
There is a private heap space in Python that contains all the Python objects and data structures. In CPython there is a memory manager responsible for managing the heap space. There are different components in Python memory manager that handle segmentation, sharing, caching, memory pre-allocation etc. Python memory manager also takes care of garbage collection by using a reference counting algorithm.
21. How are arguments passed in a Python method? By value or by reference?
Every argument in a Python method is an Object. All the variables in Python have reference to an Object. Therefore arguments in Python method are passed by Reference. Since some of the objects passed as reference are mutable, we can change those objects in a method. But for an Immutable object like String, any change done within a method is not reflected outside.
22. How do you perform unit testing for Python code?
unittest is a unit testing framework of Python. Unit testing means testing different components of software separately. Can you think why unit testing is important? Imagine a scenario, you are building software which uses three components namely A, B, and C. Now, suppose your software breaks at a point time. How will you find which component was responsible for breaking the software? Maybe it was component A that failed, which in turn failed component B, and this actually failed the software. There can be many such combinations. This is why it is necessary to test each and every component properly so that we know which component might be highly responsible for the failure of the software.
23. What is the use of generator in Python?
We can use generator to create iterators in Python. A generator is written like a regular function. lt can make use of the yield statement to return data during the function call. In this way we can write complex logic that works as an iterator. A generator is more compact than an iterator due to the fact that __iter__() and next() functions are automatically created in a generator. Also within a generator, local variables and execution state are saved between multiple calls. Therefore, there is no need to add extra variables like self.index etc. to keep track of iterations. Generator also increases the readability of the code written in Python. lt is a very simple implementation of an iterator.
24. What is the significance of functions that start and end with _ symbol in Python?
Python provides many built-in functions that are surrounded by _ symbol at the start and end of the function name. The double _ symbol is used for reserved names of functions. These are also known as system-defined names.
25. What is the difference between xrange and range in Python?
In Python, we use range(0,10) to create a list in memory for 10 numbers. Python provides another function xrange() that is similar to range() but xrange() returns a sequence of objects instead of a list of objects. In xrange() all the values are not stored in simultaneously in memory. It is a lazy loading based function. The benefit of xrange() over range() is very minimal in regular scenarios. As of version 3.1, xrange is deprecated.
26. What is Python Flask?
Flask is a micro-framework based on Python to develop web applications. It is a very simple application framework that has many extensions to build an enterprise-level application. Flask does not provide a data abstraction layer or form validation by default. External libraries can be used on top of Flask to perform such tasks.
27. What is the use of the zip() function in Python?
The built-in function zip() can be used to aggregate all the iterable objects of an iterator. It can be used to aggregate iterable objects from two iterators as well. By using the zip() function input data from different sources can be divided into a fixed number of sets.
28. How can we retrieve data from a MySQL database?
For MySQL databases, the MySQLdb module is imported. The specific database has to be connected by passing the URL, username, password, and the name of the database. Once the connection is established, a cursor with cursor() can be opened. On an open cursor, the fetch() function can be used to execute queries and retrieve data from the database tables.
29. What is the difference between split() and slicing in Python?
A split() function works on strings to cut a large piece into smaller chunks, or sub-strings. We can specify a separator to start splitting, or it uses the space as one by default. Slicing is also a string operation for extracting a part of the string, or some part of a list.
30. How to debug a piece of code in Python?
In Python, the debugger pdb can be used for debugging code. To start debugging the following lines need to be entered on the top of a Python code.
import pdb
pdb.set_trace()
After adding these lines, the code runs in debug mode. Commands like breakpoint, step through, step into etc. can be used for debugging.
Senior Python developer interview questions
31. How will you specify source code encoding in a Python source file?
By default, every source code in Python is in UTF-8 encoding. But we can also specify our own encoding for source files. This can be done by adding the following line after “#!” in the source file.
In the line above we can replace encoding with the encoding that we want to use (e.g. ascii, latin-1 etc.).
32. How will you perform Static Analysis on a Python Script?
We can use Static Analysis tool called PyChecker for this purpose. PyChecker can detect errors in Python code. PyChecker also gives warnings for any style issues. Same other tools to find bugs in Python code are pylint and pyflakes.
33. What is a Python Decorator?
A Python Decorator is a mechanism to wrap a Python function and modify its behavior by adding more functionality to it. We can use @ symbol to call a Python Decorator function.
34. What is a namespace in Python?
A Namespace in Python is a mapping between a name and an object. lt is currently implemented as a Python dictionary. E.g. the set of built-in exception names, the set of built-in names, local names in a function. At different moments in Python, different namespaces are created. Each namespace in Python can have a different lifetime. For the list of built-in names, Namespace is created when the Python interpreter starts. When a Python interpreter reads the definition of a module, it creates a global namespace for that module. When a Python interpreter calls a function, it creates a local namespace for that function.
35. What is the difference between docstring in Python and javadoc in Java?
A docstring in Python is a string used for adding comments or summarizing a piece of code in Python. The main difference between javadoc and docstring is that docstring is available during runtime as well. Whereas, javadoc is removed from the bytecode and it is not present in .class file. We can even use Docstring comments at run time as an interactive help manual. In Python, we have to specify docstring as the first statement of a code object, just after the def or dass statement. The docstring for a code object can be accessed from the '__doc__' attribute of that object.
36. What are the different methods to copy an object in Python?
There are two ways to copy objects in Python:
Shallow copy: copy.copy() function
- It makes a copy of the file from source to destination.
- It will return a shallow copy of the parameter.
Deep copy: copy.deepcopy() function
- It also produces the copy of an object from the source to destination.
- It will return a deep copy of the parameter that you can pass to the function.
37. What is a metaclass in Python?
A metaclass is the class of a class. A class defines how an instance of the class (i.e. an object) behaves while a metaclass defines how a class behaves. A class is an instance of a metaclass. One of the most common metaclass in Python is type. A metaclass is most commonly used as a class-factory.
38. What is the use of frozenset in Python?
A frozenset is a collection of unique values in Python. frozenset is an immutable collection of distinct hashable objects. Once the values in a frozenset are set, they cannot be changed. frozenset is immutable and thus, hashable, and can be used as a dictionary key or as an element of another set. Methods from set on frozenset cannot be used and updated.
39. How to check if a class is a subclass of another class?
Python provides a useful method issubclass(a,b) to check if class a is a subclass of b.
E.g. int is not a subclass of long
>>> issubclass(int,long)
False
E.g. bool is a subclass of int
>>> issubclass(bool,int)
True
40. What is the difference between “is” and “==” in Python?
“is” is used to check an object against its identity, whereas “==” is used to check the equality of two objects.
E.g.
>>> lst=[10,20,20]
>>> lst==lst[:]
True
>>> lst is lst[:]
False
Tips for acing your next interview
By refreshing your knowledge with the Python coding interview questions above for your next developer job interview, you are bound to do great! However, keep in mind that your expertise is only one part of a job interview, be yourself, keep calm and relaxed, and kick some technical interview ass.
What’s a Rich Text element?
The rich text element allows you to create and format headings, paragraphs, blockquotes, images, and video all in one place instead of having to add and format them individually. Just double-click and easily create content.
Static and dynamic content editing
A rich text element can be used with static or dynamic content. For static content, just drop it into any page and begin editing. For dynamic content, add a rich text field to any collection and then connect a rich text element to that field in the settings panel. Voila!
How to customize formatting for each rich text
Headings, paragraphs, blockquotes, figures, images, and figure captions can all be styled after a class is added to the rich text element using the "When inside of" nested selector system.
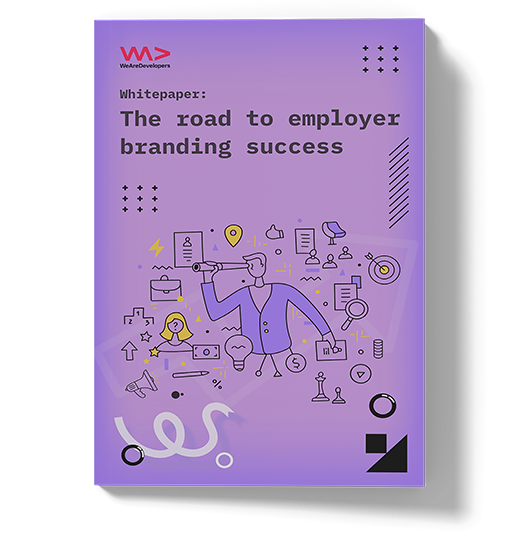